Table 17.1. JavaScript's Math Functionality
Function | Description |
---|
abs | Absolute value |
sin, cos, tan | Standard trigonometric functions; arguments in radians |
acos, asin, atan | Inverse trigonometric functions; return values in radians |
exp, log | Exponential and natural logarithm, base e |
ceil | Returns least integer greater than or equal to argument |
floor | Returns greatest integer less than or equal to argument |
min | Returns lesser of two arguments |
max | Returns greater of two arguments |
pow | Exponential; first argument is base, second is exponent |
round | Rounds argument to nearest integer |
sqrt | Square root |
1. |
javascript:var evl,em,expr=prompt ('Formula...(eg: 2*3 + 7/8)','');
This line sets up two variables and then prompts the user for an expression or formula, as shown in Figure 17.22, which is stored in expr.
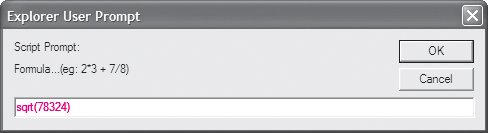
|
2. |
The next few lines need to be evaluated using JavaScript's built-in Math routines. The with(Math) line tells the interpreter that when any of these functions are seen, to evaluate them as Math commands.
The try{} warns JavaScript that what we're doing may well fail, and if so, don't panic. In fact, don't even put up an error message if there's a problem, as we'll be handling the errors ourselves.
|
| |
3. |
evl=parseFloat(eval(expr));
Evaluate the expression, and turn it into a floating-point number, which is then stored in evl.
|
4. |
If the resulting value in evl is not a number ( NaN), do the following line.
|
5. |
{throw Error('Not a number!');}
If we're here, for some reason what the user entered didn't work out to a number. When that happens we want to force an error message of "Not a number!" to display. Here's where the message is set; it will be displayed in step 7.
|
6. |
void(prompt('Result:',evl));
Otherwise, the expression was valid, so display the result, as shown in Figure 17.23.
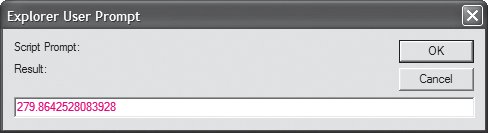
|
7. |
Here's the end of that try{} block that started in step 2. To get here, one of two things happened: either we ran into the error in step 5, or some other error entirely occurred. Either way, we "catch" the error that was "thrown" and put it up on the screen in an alert.
|