Introduction to JavaBeans
JavaBeans are Java objects developed using specific syntax rules that allow a reusable component architecture. That is, JavaBeans are reusable application components used as either GUI components or, as in our case, JSP helper classes. For more details about JavaBeans and the 1.0 Specification, refer to http://java.sun.com/products/javabeans/. JavaBeans generally provide support for
Introspection—
Introspection enables runtime determination of supported events, methods, and properties, so that tools built to the specification can determine how the bean works. Low-level reflection is used to query methods and deduce (using conventional names and type signatures) what properties, events, and public methods are supported. If the developer provides a BeanInfo class, that class is used to determine the bean's behavior. A bean Introspector class is furnished to provide a standardized methodology of introspecting different beans.
Customization—
Customization, which is applicable to GUI beans, enables developers to customize a bean's appearance and behavior so that application builders built to specification can modify it. Customization is implemented in two ways: GUI property sheets and Customizer classes. GUI beans can export a set of properties. These properties can be used to create a GUI property sheet, which lists the properties and provides an editor for each property. This property editor can then be used to update bean properties. Customizer classes are AWT components that provide the GUI behavior required to control target beans.
Events—
Events are applicable to GUI beans. They're constructs that convey state change information that can be used to connect beans. Events are conveyed from source Objects to target listener Objects via method invocations originating from the target listener. Each type of event notification is defined by a unique method. Those methods are grouped within EventListener interfaces. Event state objects are used to encapsulate the state associated with a particular event. Custom adapter classes may be used in situations where listeners cannot implement a desired interface.
Persistence—
Saving and restoring object state is also used to support customization in application builders to allow persistence of object state. JavaBeans must support the Java object serialization APIs, which include externalization. If a JavaBean doesn't implement the hidden state attribute, third-party tools may be used to restore the state of a serialized JavaBean; otherwise, Java object serialization must be used to save and restore the JavaBean's state.
Properties—
Also known as bean attributes, properties are used to support customization and developer implementation. Properties are the only feature required for non-GUI JavaBean implementations. Further discussion of properties follows.
For use within JSP pages, JavaBeans merely must define properties and implement the accessor methods (getter/setter) associated with those properties. A JavaBean must also implement at least a no-parameter (default) constructor. Properties are discrete attributes of the bean. Attributes may be built-in Java data types or user-defined data types. An attribute must be declared private, and public accessor methods must be defined for each attribute. These methods are of the form setAttributeName(attribute_data_type) and getAttributeName(). As presented in Chapter 15, "Writing WebLogic Server JavaServer Pages," to implement JavaBeans within a JSP page, you use the useBean, getProperty, and/or setProperty actions. For example, using the ItemHolderBean implemented for GAMS to create an item with the following attributes, we can use the following code:
<jsp:useBean id="item" class="com.gams.javaBeans.item.ItemHolderBean" scope="session"/>
<jsp:setProperty name="item" property="itemId" value="3004"/>
<jsp:setProperty name="item" property="title" value="Oriental Rug"/>
<jsp:setProperty name="item" property="currentBid" value="875.00"/>
<jsp:setProperty name="item" property="endDateTime" value="102,10,31"/>
To dereference bean attributes, use can use the following code:
<jsp:getProperty name="item" property="itemId" value="3004"/>
or
<%=item.getItemId()%>
For information about using JavaBeans within JSP pages, refer to the discussion of the useBean action in Chapter 15. Listing 16.1 presents a partial code listing for the ItemHolderBean.java JavaBean. As you can see, the bean definition for a JSP helper class is pretty straightforward. The bean attributes are defined in lines 8–21. In line 23, the requisite default constructor is defined.
Listing 16.1 ItemHolderBean.java
1 package com.gams.javaBeans.item;
2
3 import java.io.Serializable;
4 import java.sql.Date;
5
6 public class ItemHolderBean implements Serializable
7 {
8 private Integer categoryId;
9 private Float currentBid;
10 private String description;
11 private Date endDateTime;
12 private boolean isFeatured;
13 private Boolean isOpen;
14 private Integer itemId;
15 private long minimumBid;
16 private String pictureFile;
17 private Integer quantityAvailable;
18 private Float reservePrice;
19 private Integer sellerId;
20 private Date startDateTime;
21 private String title;
22
23 public ItemHolderBean()
24 {
25 }
26 public ItemHolderBean(Integer itId, String title, Float curBid, Date endDate )
27 {
28 itemId = itId;
29 title = title;
30 currentBid = curBid;
31 endDateTime= endDate;
32 }
33 public ItemHolderBean(Integer integer, Float float1, String s, Date date, boolean
flag, Boolean boolean1, Integer integer1,
34 long l, String s1, Integer integer2, Float float2, Integer integer3, Date date1,
35 String s2)
36 {
37 categoryId = integer;
38 currentBid = float1;
39 description = s;
40 endDateTime = date;
41 isFeatured = flag;
42 isOpen = boolean1;
43 itemId = integer1;
44 minimumBid = l;
45 pictureFile = s1;
46 quantityAvailable = integer2;
47 reservePrice = float2;
48 sellerId = integer3;
49 startDateTime = date1;
50 title = s2;
51 }
52
53
54 public Integer getCategoryId()
55 {
56 return categoryId;
57 }
58
59 public void setCategoryId(Integer integer)
60 {
61 categoryId = integer;
62 }
63
64 public Float getCurrentBid()
65 {
66 return currentBid;
67 }
68
69 public void setCurrentBid(Float float1)
70 {
71 currentBid = float1;
72 }
73
74 public String getDescription()
75 {
76 return description;
77 }
78
79 public void setDescription(String s)
80 {
81 description = s;
82 }
83
84 public Date getEndDateTime()
85 {
86 return endDateTime;
87 }
88
89 public void setEndDateTime(Date date)
90 {
91 endDateTime = date;
92 }
93
94 public boolean getIsFeatured()
95 {
96 return isFeatured;
97 }
98
99 public void setIsFeatured(boolean flag)
100 {
101 isFeatured = flag;
102 }
103
104 public Boolean getIsOpen()
105 {
106 return isOpen;
107 }
108
109 public void setIsOpen(Boolean boolean1)
110 {
111 isOpen = boolean1;
112 }
113
114 public Integer getItemId()
115 {
116 return itemId;
117 }
118
119 public void setItemId(Integer integer)
120 {
121 itemId = integer;
122 }
123
124 public long getMinimumBid()
125 {
126 return minimumBid;
127 }
128
129 public void setMinimumBid(long l)
130 {
131 minimumBid = l;
132 }
133
134 public String getPictureFile()
135 {
136 return pictureFile;
137 }
138
139 public void setPictureFile(String s)
140 {
141 pictureFile = s;
142 }
143
144 public Integer getQuantityAvailable()
145 {
146 return quantityAvailable;
147 }
148
149 public void setQuantityAvailable(Integer integer)
150 {
151 quantityAvailable = integer;
152 }
153
154 public Float getReservePrice()
155 {
156 return reservePrice;
157 }
158
159 public void setReservePrice(Float float1)
160 {
161 reservePrice = float1;
162 }
163
164 public Integer getSellerId()
165 {
166 return sellerId;
167 }
168
169 public void setSellerId(Integer integer)
170 {
171 sellerId = integer;
172 }
173
174 public Date getStartDateTime()
175 {
176 return startDateTime;
177 }
178
179 public void setStartDateTime(Date date)
180 {
181 startDateTime = date;
182 }
183
184 public String getTitle()
185 {
186 return title;
187 }
188
189 public void setTitle(String s)
190 {
191 title = s;
192 }
193 }
194
Two constructors (one a 4 parameter and the other a 14 parameter; used to construct ItemBeanHolder Java objects) are defined in lines 26–51. The remainder of the code (lines 54–192) defines attribute getter and setter methods. Listing 16.2 displays a simple JSP, viewItemHolderBean.jsp, which uses the ItemHolderBean.
Listing 16.2 viewItemHolderBean.jsp
1 <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
2
3 <html>
4 <head>
5 <title>Global Auctions - View Item Holder Bean</title>
6 </head>
7
8 <body><h1>View Item Holder Bean</h1>
9 <jsp:useBean id="item" class="com.gams.javaBeans.item.ItemHolderBean" scope="session"/>
10 <jsp:setProperty name="item" property="itemId" value="3003"/>
11 <jsp:setProperty name="item" property="title" value="Oriental Rug"/>
12 <jsp:setProperty name="item" property="currentBid" value="875.00"/>
13 <table>
14 <tr><td>Item Number: </td><td><%=item.getItemId()%></td></tr>
15 <tr><td>Description: </td><td><%=item.getTitle()%></td></tr>
16 <tr><td>Current Bid: </td>
17 <td><jsp:getProperty name="item" property="currentBid" /></td>
18 </tr>
19 </table>
20 </body>
21 </html>
Note the declaration of the JavaBean item in line 9. Lines 10–12 implement <jsp:setProperty> actions, which set attributes on the item bean. Lines 14 and 15 dereference the item bean attributes itemId and title using Java standard method call syntax. Note line 17 where the <jsp:getProperty> action is used to dereference attribute currentBid. A browser display of viewItemHolderBean.jsp is provided in Figure 16.3.
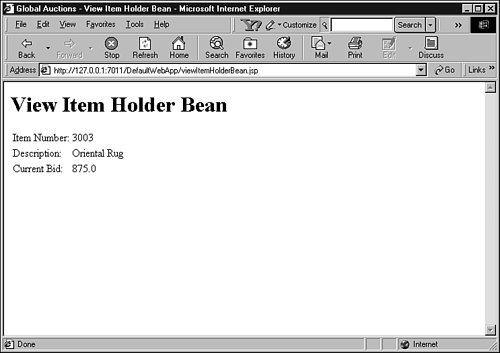
|