The HttpSession Class
The HttpSession stores information between browser requests. In addition to storing and retrieving objects, you can control when the session is created and how long the session stays active after there has been no activity.
Storing and Retrieving Objects
You can store and retrieve objects in the session that will be available the next time the browser sends a request. Use the getAttribute, setAttribute, and removeAttribute methods to store and retrieve the objects:
public Object getAttribute(String name)
public void setAttribute(String name, Object value)
public void removeAttribute(String name)
You can get a list of available objects by calling getAttributeNames:
public Enumeration getAttributeNames()
Same Method Names, Different Classes 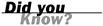 | In case you haven't noticed, the classes that allow you to store objects with application, session, request, and page scope (ServletContext, HttpSession, ServletRequest, and PageContext) all use the same methods to get, store, and remove attributes. |
To make it easier to get to the servlet context that this session is associated with, HttpSession provides the method getServletContext:
public javax.servlet.ServletContext getServletContext()
Controlling Session Termination
One of the difficulties in dealing with sessions is that the server never knows for sure that the session has ended unless you create some sort of logout mechanism. Because the server and the browser spend most of their time disconnected from each other, the server has no way to know that the user has closed the browser window and is now deeply involved in a game of EverQuest.
Putting a logout mechanism into your Web pages is a great idea if your Web site has the notion of login and logout. When the user logs out, you just invalidate the session by calling the invalidate method:
public void invalidate()
Even with a logout mechanism, though, you can't be sure that the user will be kind enough to actually log out before shutting down the browser. Whether or not you have a logout mechanism, you can force sessions to terminate after a specified period of inactivity. Typical servlet and JSP engines set the timeout at 30 minutes to begin with. You can usually change the default timeout for all sessions with the server's administration tools, or you can query and change the session timeout by calling getMaxInactiveInterval and setMaxInactiveInterval:
public int getMaxInactiveInterval()
public void setMaxInactiveInterval(int interval)
Getting Session Status
There are a few aspects of the session that you might be interested in. First, you can get the unique identifier of the session by calling getId:
public String getId()
This identifier is unique across all the sessions within your servlet engine, but not necessarily unique if you are running multiple servlet engines. If you are using only a single servlet engine, you can pass this identifier to other parts of your application (CORBA services, Enterprise JavaBeans) to serve as a unique identifier for any session-oriented data stored elsewhere.
If you use the session ID in other parts of your application, you are limiting yourself to using only a single servlet engine. To allow for the possibility of multiple servlet and JSP engines passing session identifiers to other portions of your application, consider putting some unique prefix in front of the session ID before you pass it to another module. For example, you might have an "Internet customer" JSP engine with a consumer-friendly interface. You might also have an "Internal-only" servlet engine that handles internal company data. If both of these engines need to access the same service and pass session IDs, you might append "Net" before the Internet customer session IDs and "Int" before the internal customer session IDs.
The isNew method tells you whether a session has just been created:
public boolean isNew()
If a session is new, none of the session information has been sent to the client browser. Remember, the only part of the session that is sent to the browser is the session ID, which may be stored in a cookie.
You can find out when a session was created by calling getCreationTime:
public long getCreationTime()
The time value returned by getCreationTime is the number of milliseconds since January 1, 1970. You can create a date object from the creation time by passing the time value to the Date constructor like this:
Date creationTime = new Date(session.getCreationTime());
The getLastAccessedTime method returns the most recent time that a client browser with this session accessed the server:
public long getLastAccessedTime()
As with the creation time, the last access time value is the number of milliseconds since January 1, 1970; you can create a Date object from this time value by passing the value to the Date constructor. The last access time is the value the servlet engine uses to determine when a session has timed out.
Accessing Session Variables Does Not Reset the Session Timer 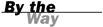 | Accessing session variables does not change the last access time. The only thing that updates the time is an incoming request from a browser. In other words, you cannot prevent a servlet from timing out by accessing a session variable. |
|